- Java Development Kit, from Oracle site
- ADT Bundle, from Android Developer site, unzip it (includes eclipse)
- Open up Eclipse from the eclipse folder.
- Go to Help menu and Install New Software...
- In Work with:, enter site: http://dist.springsource.com/release/TOOLS/gradle
- Download Eclipse Integration Gradle.
- From http://libgdx.badlogicgames.com/download.html, download setup app (a jar file).
After clicking on Generate and getting the successful message, these are the contents:
Now I can open eclipse included in the ADT bundle, and select File -> Import Grade > Import Gradle Project and select the files:
Running the file right now, right clicking on the desktop package and running as Java application, we get:
This is the default java file displaying a Texture:
package com.tutorial.helloGame; import com.badlogic.gdx.ApplicationAdapter; import com.badlogic.gdx.Gdx; import com.badlogic.gdx.graphics.GL20; import com.badlogic.gdx.graphics.Texture; import com.badlogic.gdx.graphics.g2d.SpriteBatch; public class HellloGame extends ApplicationAdapter { SpriteBatch batch; Texture img; @Override public void create () { batch = new SpriteBatch(); img = new Texture("badlogic.jpg"); } @Override public void render () { Gdx.gl.glClearColor(1, 0, 0, 1); Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT); batch.begin(); batch.draw(img, 0, 0); batch.end(); } }
This is the HelloWorld program using the BitmapFont:
package com.tutorial.helloGame; import com.badlogic.gdx.ApplicationAdapter; import com.badlogic.gdx.Gdx; import com.badlogic.gdx.graphics.GL20; import com.badlogic.gdx.graphics.OrthographicCamera; import com.badlogic.gdx.graphics.g2d.BitmapFont; import com.badlogic.gdx.graphics.g2d.SpriteBatch; public class HellloGame extends ApplicationAdapter { OrthographicCamera camera; SpriteBatch batch; BitmapFont font; float w,h; @Override public void create () { w = Gdx.graphics.getWidth(); // width of screen h = Gdx.graphics.getHeight(); // height of screen camera = new OrthographicCamera(); // 2D camera camera.setToOrtho(false, w, h); // y increases upwards, viewport = window batch = new SpriteBatch(); // batch drawing font = new BitmapFont(); // no parameters - thus default 15-pt Arial font.setColor(0.0f, 0.0f, 1.0f, 1.0f); // blue font font.setScale(5); // font scaled up } @Override public void render () { Gdx.gl.glClearColor(1, 1, 0, 1); // Clear color is yellow Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT); // Clear buffer with Clear color batch.setProjectionMatrix(camera.combined); // Set Projection Matrix batch.begin(); // begin drawing font.draw(batch, "Hello World", w/2-180, h/2+50); // Draw the Hello World text batch.end(); // end drawing } @Override public void dispose() { batch.dispose(); // remove batch when app ending font.dispose(); // remove font when app ending } }
Result:
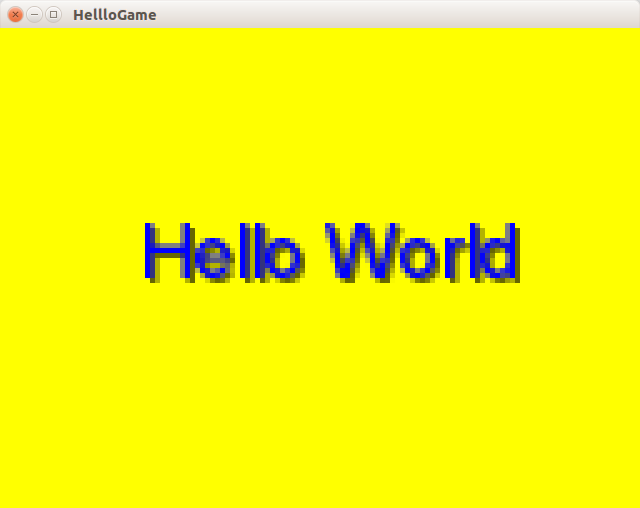
Very useful, thanks a lot. A little offer is that give explanation of your code.
ReplyDeleteFor example; "OrthographicCamera is to be used in 2D environments only as it implements a parallel (orthographic) projection and there will be no scale factor for the final image regardless where the objects are placed in the world."
I took this definition on LibGDX github wiki :)